前回は平面検出時に生成されたプレーンの表示切替とオブジェクトの全消去を実装しました
今回は選択したオブジェクトを動かすことにチャレンジしてみようと思います
スクリプト
オブジェクトを動かすには、現在選択されているオブジェクトの座標をスワイプやボタン等なんらかの入力で変更する必要があります
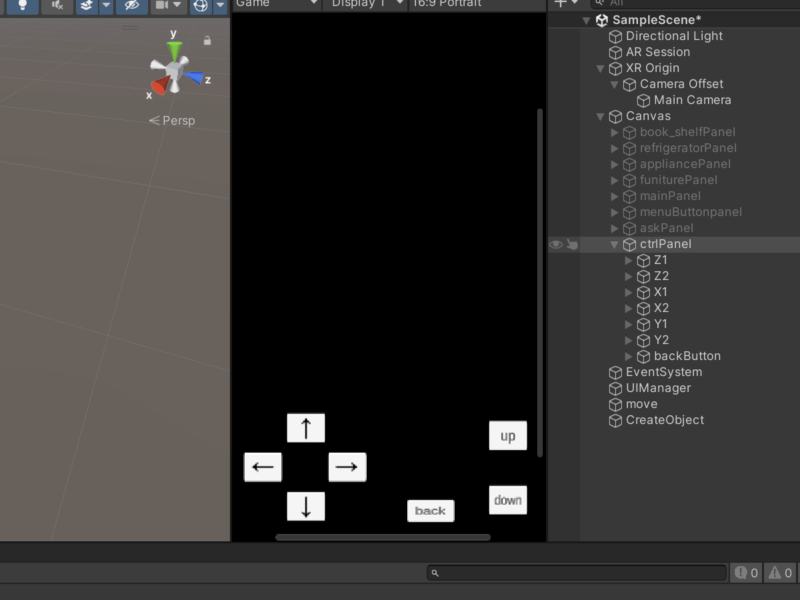
このようなパネルを作り
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.EventSystems;
public class move : MonoBehaviour
{
public CreateObject createObject;
public UIManager uimanager;
[SerializeField] EventSystem eventSystem;
private GameObject selectedObj;
public void Getobj()
{
if (Input.GetMouseButton(0))
{
try
{
selectedObj = eventSystem.currentSelectedGameObject.gameObject;
Debug.Log("オブジェクト名=" + selectedObj);
}
catch //(NullReferenceException ex)
{ }
}
}
public void Z1()
{
//Debug.Log("Aobjnow=" + uimanager.Aobjnow);
//Debug.Log("Fobjnow=" + uimanager.Fobjnow);
//Debug.Log("オブジェクト名=" + createObject.selectedObj);
selectedObj.transform.position += new Vector3(0, 0, 1 * Time.deltaTime);
}
public void Z2()
{
selectedObj.transform.position += new Vector3(0, 0, -1 * Time.deltaTime);
}
public void X1()
{
selectedObj.transform.position += new Vector3(1, 0, 0 * Time.deltaTime);
//Debug.Log("オブジェクト名=" + createObject.selectedObj);
}
public void X2()
{
selectedObj.transform.position += new Vector3(-1, 0, 0 * Time.deltaTime);
}
public void Y1()
{
selectedObj.transform.position += new Vector3(0, 1, 0 * Time.deltaTime);
//Debug.Log("オブジェクト名=" + createObject.selectedObj);
}
public void Y2()
{
selectedObj.transform.position += new Vector3(0, -1, 0 * Time.deltaTime);
}
}
このようにボタン制御用のスクリプトを用意してみました
しかしオブジェクトをタップした時点ではその情報を取得しているように見えるのですが、いざ座標を変更しようとすると何も選択されていないとエラーが返って来てしまいます
色々調べた結果、これは「変数のスコープ」(定義された場所によって呼び出せる範囲が変わる)という仕組みが関わっていることが分かりました
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.XR.ARFoundation;
using UnityEngine.XR.ARSubsystems;
using UnityEngine.EventSystems;
public class CreateObject2 : MonoBehaviour
{
public GameObject selectedObj;
public GameObject clickedGameObject;
public List<GameObject> fObjects = new List<GameObject>();
public List<GameObject> aObjects = new List<GameObject>();
public UIManager uimanager;
private ARRaycastManager raycastManager;
private List<ARRaycastHit> hitResults = new List<ARRaycastHit>();
void Awake()
{
raycastManager = GetComponent<ARRaycastManager>();
}
void Update()
{
Vector3? touchPosition = null;
if (Input.GetMouseButtonDown(0) && !(EventSystem.current.IsPointerOverGameObject()))
{
touchPosition = Input.GetTouch(0).position;
}
if (touchPosition != null && Hit1((Vector3)touchPosition) && uimanager.Aobjnow > 9000 && fObjects != null)
{
try
{
ObjChanged();
}
finally { }
}
if (touchPosition != null && Hit2((Vector3)touchPosition))
{
Debug.Log("オブジェクト 名:" + clickedGameObject);
}
if (touchPosition != null && Hit3((Vector3)touchPosition))
{
uimanager.menupanels[3].SetActive(false);
uimanager.menupanels[5].SetActive(true);
Debug.Log("オブジェクト 名:" + clickedGameObject);
selectedObj = clickedGameObject;
}
}
public void ObjChanged()
{
switch (uimanager.Aobjnow)
{
case int n when (n > 9000):
Debug.Log("Fobjnow=" + uimanager.Fobjnow);
Debug.Log("オブジェクト名:" + fObjects[uimanager.Fobjnow]);
clickedGameObject = fObjects[uimanager.Fobjnow];
uimanager.Aobjnow = 8000;
break;
default:
break;
}
switch (uimanager.Fobjnow)
{
case int n when (n > 9000):
Debug.Log("Aobjnow=" + uimanager.Aobjnow);
Debug.Log("オブジェクト名:" + aObjects[uimanager.Aobjnow]);
clickedGameObject = aObjects[uimanager.Aobjnow];
uimanager.Fobjnow = 8000;
break;
default:
break;
}
}
bool Hit1(Vector3 touchPosition)
{
if (touchPosition != null && !(EventSystem.current.IsPointerOverGameObject()))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);//クリックした場所にレイを飛ばす
RaycastHit hit = new RaycastHit();
if (Physics.Raycast(ray, out hit) && hit.collider.gameObject.CompareTag("arplane"))
{
return true;
}
}
return false;
}
bool Hit2(Vector3 touchPosition)
{
if (touchPosition != null && !(EventSystem.current.IsPointerOverGameObject()))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);//クリックした場所にレイを飛ばす
RaycastHit hit = new RaycastHit();
if (Physics.Raycast(ray, out hit) && !hit.collider.gameObject.CompareTag("arplane")
&& hit.collider.gameObject != clickedGameObject && hit.collider.gameObject.CompareTag("d_obj"))
{
clickedGameObject = hit.collider.gameObject;
return true;
}
}
return false;
}
bool Hit3(Vector3 touchPosition)
{
if (touchPosition != null && !(EventSystem.current.IsPointerOverGameObject()))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);//クリックした場所にレイを飛ばす
RaycastHit hit = new RaycastHit();
if (Physics.Raycast(ray, out hit) && !hit.collider.gameObject.CompareTag("arplane")
&& hit.collider.gameObject == clickedGameObject && hit.collider.gameObject.CompareTag("d_obj"))
{
clickedGameObject = hit.collider.gameObject;
return true;
}
}
return false;
}
}
CreateObjectスクリプトをこのように別の方式に書き換えてみました
ヒット判定を3つに分けて、タップした時の条件と合えばそれぞれの命令を実行するというものですが
実行してみるとやはりオブジェクト情報の受け渡しができておらずうまくいきませんでした・・・
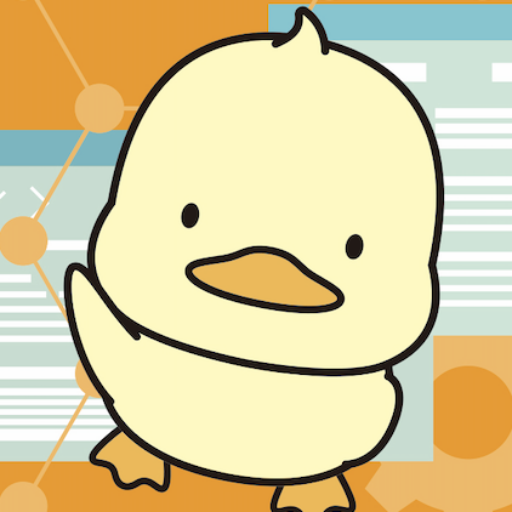
もふもふ
むずいもふ(; ・`д・´)
取得したオブジェクト情報をreturnで拾おうと試みましたが、そもそもvoidメソッドは戻り値がないので無理ですと断られてしまいました
お手上げ状態なので、とりあえずアップデート内で座標に干渉してみる事にしました
using System.Collections;
using System.Collections.Generic;
using Unity.VisualScripting;
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.InputSystem.HID;
using UnityEngine.XR.ARFoundation;
using UnityEngine.XR.ARSubsystems;
using UnityEngine.UI;
using UnityEngine.UIElements;
public class CreateObject : MonoBehaviour
{
[SerializeField] EventSystem eventSystem;
public GameObject selectedObj;
public GameObject clickedGameObject;
public List<GameObject> fObjects = new List<GameObject>();
public List<GameObject> aObjects = new List<GameObject>();
public UIManager uimanager;
private Vector3 mouse;
private ARRaycastManager raycastManager;
private List<ARRaycastHit> hitResults = new List<ARRaycastHit>();
void Awake()
{
eventSystem = EventSystem.current;
raycastManager = GetComponent<ARRaycastManager>();
}
public void Update()
{
mouse = Input.mousePosition;
//if (EventSystem.current.IsPointerOverGameObject(Input.GetTouch(0).fingerId)) //スマホ入力
if (EventSystem.current.IsPointerOverGameObject()) return;//UIをタップした場合飛ばす
{
if (Input.GetMouseButton(0))
//if (Input.touchCount > 0 && Input.GetTouch(0).phase == TouchPhase.Began)
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);//クリックした場所にレイを飛ばす
RaycastHit hit = new RaycastHit();
if (Physics.Raycast(ray, out hit) != false)
{
if (hit.collider.gameObject.CompareTag("arplane") && uimanager.Aobjnow > 9000)
{
//clickedGameObject = fObjects[uimanager.Fobjnow];
Debug.Log("Fobjnow=" + uimanager.Fobjnow);
Debug.Log("オブジェクト名:" + fObjects[uimanager.Fobjnow]);
Instantiate(fObjects[uimanager.Fobjnow], hit.point, Quaternion.Euler(0, 90, 0));
uimanager.Aobjnow = 8000;
}
else if (hit.collider.gameObject.CompareTag("arplane") && uimanager.Fobjnow > 9000)
{
//clickedGameObject = aObjects[uimanager.Aobjnow];
Debug.Log("Aobjnow=" + uimanager.Aobjnow);
Debug.Log("オブジェクト名:" + aObjects[uimanager.Aobjnow]);
Instantiate(aObjects[uimanager.Aobjnow], hit.point, Quaternion.Euler(0, 90, 0));
uimanager.Fobjnow = 8000;
}
//もしレイが何かのコライダーに衝突し、かつそのコライダーのタグがd_objで、かつclickedGameObjectと異なる場合
if (Physics.Raycast(ray, out hit) && !hit.collider.gameObject.CompareTag("arplane") && hit.collider.gameObject != clickedGameObject && hit.collider.gameObject.CompareTag("d_obj"))
{
clickedGameObject = hit.collider.gameObject;
Debug.Log("オブジェクト 名:" + clickedGameObject);
}
//もしレイが何かのコライダーに衝突し、かつそのコライダーのタグがd_objで、かつclickedGameObjectと同じ場合
else if (Physics.Raycast(ray, out hit) && !hit.collider.gameObject.CompareTag("arplane") && hit.collider.gameObject == clickedGameObject && hit.collider.gameObject.CompareTag("d_obj"))
{
Debug.Log("オブジェクト 名:" + clickedGameObject);
clickedGameObject = hit.collider.gameObject;
clickedGameObject.transform.position = Camera.main.ScreenToWorldPoint(new Vector3(mouse.x, y, 2))
}
}
}
}
}
}
一番最後の行でオブジェクトの位置を変更しようとしています
条件をクリアして選択されたオブジェクトをスワイプで動かす方式です
実行してみると・・・
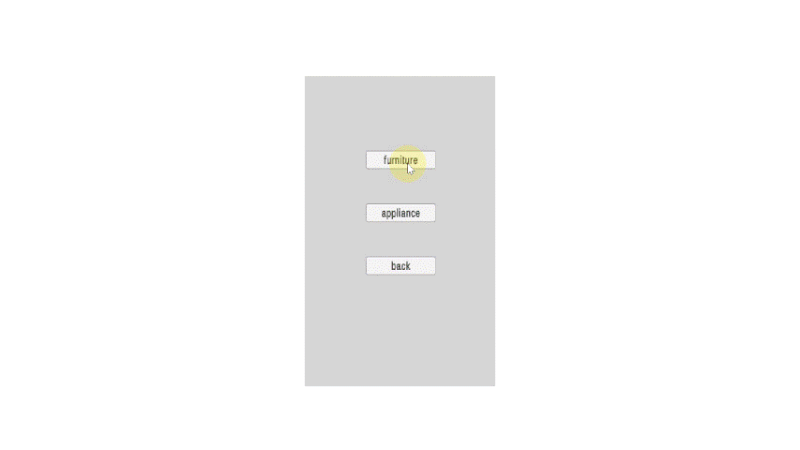
選択した物を動かすという目的は果たせているのですが、動きがヤバいです・・・
しかもこの手法ではZ方向に動きません・・・
まとめ
1歩前進は出来たものの、まだまだ改善が必要なので引き続き理解度を深めていこうと思います